Introduction
Measuring distance using an HC-SR04 Ultrasonic sensor module and posting its status on an OLED SSD1306 White 128X64 IIC I2C Serial Display Module using an Arduino UNO microcontroller is a system that uses an HC-SR04 ultrasonic sensor to detect the distance of an object in centimeters and an OLED SSD1306 display to show the distance value
The HC-SR04 sensor emits an ultrasonic sound wave and measures the time it takes for the sound wave to be reflected back by an object. The distance of the object is then calculated by dividing the time by the speed of sound. The OLED SSD1306 display is an IIC/I2C serial display module that is connected to the Arduino UNO microcontroller via the I2C bus and used to display the distance value in centimeters.
Hardware Components
You will require the following hardware for Ultrasonic Sensor OLED with Arduino.
S.no | Component | Value | Qty |
---|---|---|---|
1. | Arduino UNO | – | 1 |
2. | USB Cable Type A to B | – | 1 |
3. | I2C OLED Display 128×64 | SSD1306 | 1 |
4. | Ultrasonic Sensor | – | 1 |
5. | 9V Power Adapter for Arduino | – | 1 |
6. | Breadboard | – | 1 |
7. | Jumper Wires | – | 1 |
Ultrasonic Sensor OLED with Arduino
- Connect the HC-SR04 ultrasonic sensor to the Arduino using the trigger and echo pins specified in the sensor’s documentation. Connect the OLED SSD1306 display to the Arduino using the SDA and SCL pins, and the specified address.
- In the Arduino IDE, include the necessary libraries for the HC-SR04 sensor and OLED display.
#include <Wire.h>
#include <Adafruit_SSD1306.h>
#include <Adafruit_Sensor.h>
- In the setup() function, initialize the serial communication, the OLED display, and the HC-SR04 sensor:
Serial.begin(9600);
display.begin(SSD1306_SWITCHCAPVCC, 0x3C); // Initialize OLED display
pinMode(trigPin, OUTPUT);
pinMode(echoPin, INPUT);
- In the loop() function, measure the distance using the HC-SR04 sensor:
distance = measureDistance();
- Clear the OLED display, set the cursor position, and print the distance value on it:
display.clearDisplay();
display.setCursor(0,0);
display.print("Distance: ");
display.print(distance);
display.println(" cm");
display.display();
- Send the distance value to the serial monitor using the Serial.println() function:
Serial.print("Distance: ");
Serial.print(distance);
Serial.println(" cm");
- (Optional) You can also create a custom function to measure the distance using the HC-SR04 sensor.
long measureDistance() {
// Send a trigger pulse to the HC-SR04 sensor
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
// Measure the duration of the echo pulse
long duration = pulseIn(echoPin, HIGH);
// Calculate the distance in centimeters
long distance = duration / 58.2;
return distance;
}
Schematic
Make connections according to the circuit diagram given below.
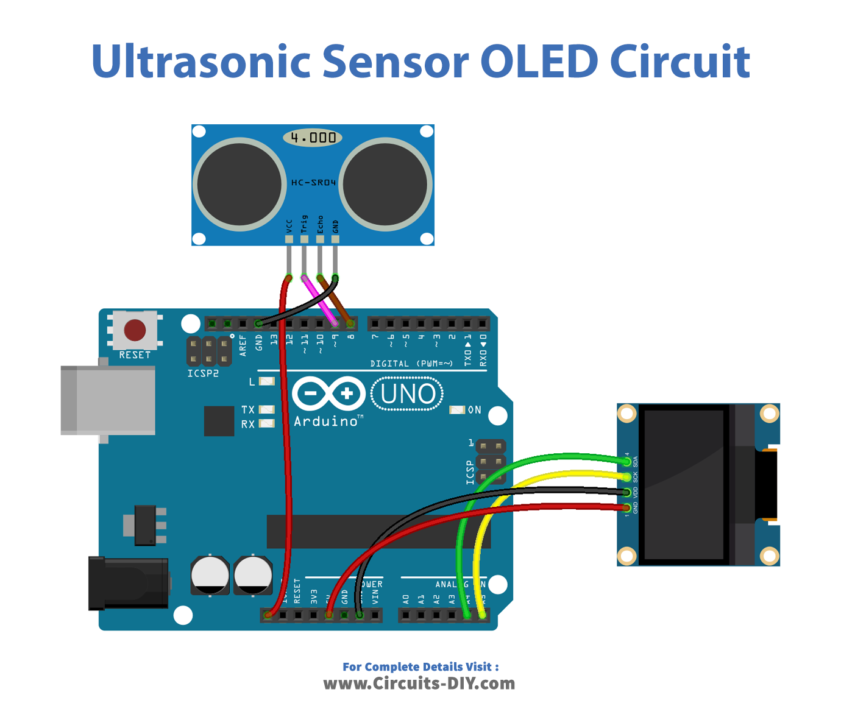
Wiring / Connections
Arduino | Ultrasonic Sensor | OLED |
---|---|---|
IODEF | VCC | |
GND | GND | GND |
D9 | TRIG | |
D8 | ECHO | |
5V | VCC | |
A4 | SCK | |
A5 | SDA |
Installing Arduino IDE
First, you need to install Arduino IDE Software from its official website Arduino. Here is a simple step-by-step guide on “How to install Arduino IDE“.
Installing Libraries
Before you start uploading a code, download and unzip the following libraries at /Progam Files(x86)/Arduino/Libraries (default), in order to use the sensor with the Arduino board. Here is a simple step-by-step guide on “How to Add Libraries in Arduino IDE“.
Code
Now copy the following code and upload it to Arduino IDE Software.
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#define SCREEN_WIDTH 128 // OLED display width, in pixels
#define SCREEN_HEIGHT 64 // OLED display height, in pixels
const int TRIG_PIN = 9; // Arduino pin connected to Ultrasonic Sensor's TRIG pin
const int ECHO_PIN = 8; // Arduino pin connected to Ultrasonic Sensor's ECHO pin
Adafruit_SSD1306 oled(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, -1); // create SSD1306 display object connected to I2C
String tempString;
void setup() {
Serial.begin(9600);
// initialize OLED display with address 0x3C for 128x64
if (!oled.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
while (true);
}
delay(2000); // wait for initializing
oled.clearDisplay(); // clear display
oled.setTextSize(2); // text size
oled.setTextColor(WHITE); // text color
oled.setCursor(0, 10); // position to display
tempString.reserve(10); // to avoid fragmenting memory when using String
}
void loop() {
// generate 10-microsecond pulse to TRIG pin
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// measure duration of pulse from ECHO pin
long duration_us = pulseIn(ECHO_PIN, HIGH);
// calculate the distance
float distance_cm = 0.017 * duration_us;
// print the value to Serial Monitor
Serial.print("distance: ");
Serial.print(distance_cm);
Serial.println(" cm");
tempString = String(distance_cm, 2); // two decimal places
tempString += " cm";
Serial.println(tempString); // print the temperature in Celsius to Serial Monitor
oledDisplayCenter(tempString); // display temperature on OLED
}
void oledDisplayCenter(String text) {
int16_t x1;
int16_t y1;
uint16_t width;
uint16_t height;
oled.getTextBounds(text, 0, 0, &x1, &y1, &width, &height);
// display on horizontal and vertical center
oled.clearDisplay(); // clear display
oled.setCursor((SCREEN_WIDTH - width) / 2, (SCREEN_HEIGHT - height) / 2);
oled.println(text); // text to display
oled.display();
}
Working Explanation
First, the necessary libraries for the HC-SR04 sensor and OLED display are included in the code. Then, the system initializes the serial communication and the OLED display in the setup() function, and sets the trigger and echo pin of the HC-SR04 sensor as output and input respectively. In the loop() function, the system uses the function measureDistance() to measure the distance using the HC-SR04 sensor, it then sends the distance value to the OLED SSD1306 display by clearing the display, setting the cursor position, and printing the distance value on it. The system also sends the distance value to the serial monitor by using the Serial.println() function.
The measureDistance() function uses digitalWrite() and delayMicroseconds() functions to send a trigger pulse to the HC-SR04 sensor, which causes the sensor to emit an ultrasonic sound wave. The function then uses the pulseIn() function to measure the duration of the echo pulse, which is the time it takes for the sound wave to travel to an object and be reflected back to the sensor. The distance to the object can be calculated by dividing the duration of the echo pulse by the speed of sound (58.2 cm/microsecond) and returning it as a distance value.
Applications
- Level Measurement
- Robotics
- Industrial Automation
- Security Systems
- Proximity Detection
- Automotive
- Medical equipment
- Home Automation
Conclusion.
We hope you have found this Ultrasonic Sensor OLED Circuit very useful. If you feel any difficulty in making it feel free to ask anything in the comment section.