Introduction
Toggling a 5V SPDT relay with a TTP223 touch sensor to run AC appliances using an Arduino UNO is a process of controlling an AC appliance using an Arduino microcontroller and an SPDT relay. The TTP223 touch sensor is used to trigger the relay to turn on or off the AC appliance.
The TTP223 touch sensor is a touch-sensitive switch that can detect a touch and output a digital signal (HIGH or LOW). It has three pins: VCC, GND, and OUT. The VCC and GND pins are used to power the touch sensor, while the OUT pin outputs a digital signal depending on whether the sensor is touched or not.
Hardware Components
You will require the following hardware for Touch Sensor Toggle Relay with Arduino.
S.no | Component | Value | Qty |
---|---|---|---|
1. | Arduino UNO | – | 1 |
2. | USB Cable Type A to B | – | 1 |
3. | Touch Sensor | – | 1 |
4. | Relay | – | 1 |
5. | Warning Light Bright Waterproof | – | 1 |
6. | Power Adapter | 12V- | 1 |
7. | DC Power Jack | – | 1 |
8. | Power Adapter for Arduino | 9V | 1 |
9. | Jumper Wires | – | 1 |
Touch Sensor Toggle Relay with Arduino
- Connect the TTP223 touch sensor to the Arduino UNO. The TTP223 has three pins, connect the VCC pin to 5V on the Arduino, the GND pin to GND on the Arduino, and the OUT pin to any digital pin on the Arduino.
- Connect the relay to the Arduino UNO. The relay has three pins, connect the VCC pin to 5V on the Arduino, the GND pin to GND on the Arduino, and the IN pin to any digital pin on the Arduino.
- In the Arduino IDE, include the default
digitalRead()
anddigitalWrite()
functions in your sketch. - In the setup() function, initialize the serial monitor with a baud rate of 9600 and configure the touch sensor pin as an input
void setup() {
Serial.begin(9600);
pinMode(2, INPUT); // 2 is the digital pin number connected to the OUT of TTP223
}
- In the loop() function, check the state of the touch sensor pin using the
digitalRead()
function and toggle the relay accordingly and display the status on the serial monitor
void loop() {
if (digitalRead(2) == HIGH) {
digitalWrite(4, !digitalRead(4)); // 4 is the pin number connected to the IN of Relay
if(digitalRead(4) == HIGH) {
Serial.println("Relay is On");
} else {
Serial.println("Relay is Off");
}
}
delay(100);
}
- Upload the code to the Arduino UNO and open the serial monitor to see the status of the relay.
Schematic
Make connections according to the circuit diagram given below.
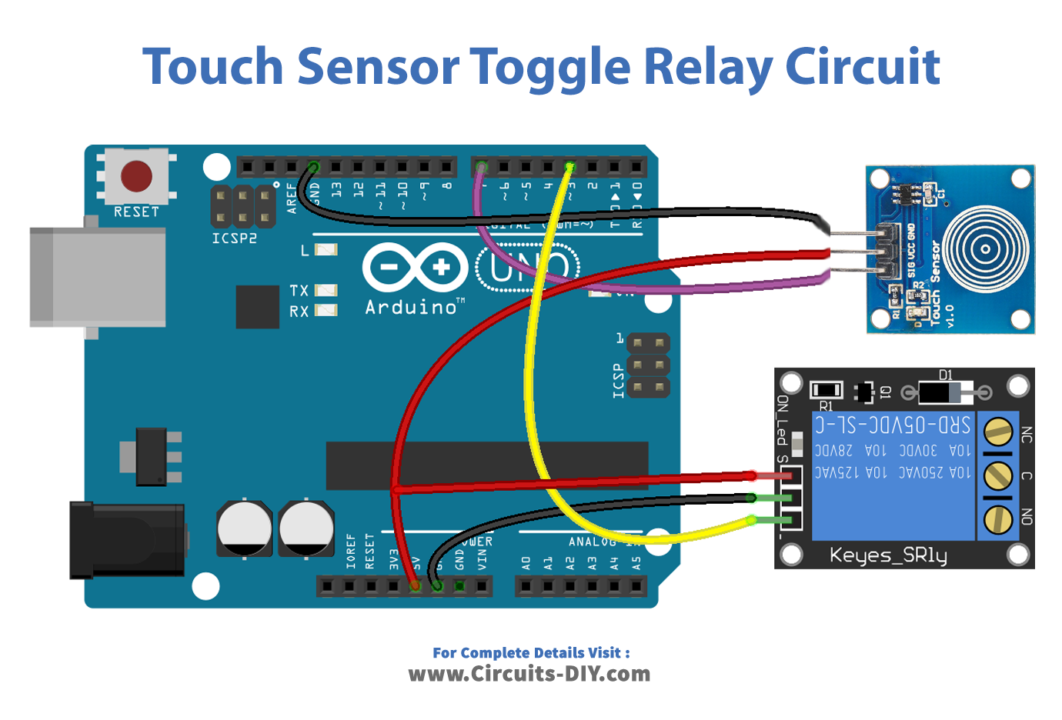
Wiring / Connections
Arduino | Touch Sensor | Relay |
---|---|---|
5V | VCC | VCC |
GND | GND | GND |
D7 | SIG PIN | |
D3 | INP |
Installing Arduino IDE
First, you need to install Arduino IDE Software from its official website Arduino. Here is a simple step-by-step guide on “How to install Arduino IDE“.
Code
Now copy the following code and upload it to Arduino IDE Software.
const int TOUCH_SENSOR_PIN = 7; // Arduino pin connected to touch sensor's pin
const int RELAY_PIN = 3; // Arduino pin connected to relay's pin
// variables will change:
int relayState = LOW; // the current state of relay
int lastTouchState; // the previous state of touch sensor
int currentTouchState; // the current state of touch sensor
void setup() {
Serial.begin(9600); // initialize serial
pinMode(TOUCH_SENSOR_PIN, INPUT); // set arduino pin to input mode
pinMode(RELAY_PIN, OUTPUT); // set arduino pin to output mode
currentTouchState = digitalRead(TOUCH_SENSOR_PIN);
}
void loop() {
lastTouchState = currentTouchState; // save the last state
currentTouchState = digitalRead(TOUCH_SENSOR_PIN); // read new state
if(lastTouchState == LOW && currentTouchState == HIGH) {
Serial.println("The sensor is touched");
// toggle state of relay
relayState = !relayState;
// control relay arccoding to the toggled state
digitalWrite(RELAY_PIN, relayState);
}
}
Working Explanation
In the setup() function, the serial monitor is initialized with a baud rate of 9600, and the touch sensor pin is configured as an input using the pinMode() function.
In the loop() function, the state of the touch sensor pin is read using the digitalRead() function. If the touch sensor is pressed, the state of the relay is toggled using the digitalWrite() function. It uses the !
operator to toggle the current state of the pin connected to the relay. The digitalRead()
function is used to check the current state of the relay pin. If the relay is on, the code will print “Relay is On” on the serial monitor, otherwise, it will print “Relay is Off”.
Applications
- Remote Control of AC Appliances
- Automatic lighting control
- Power Management
- Smart home devices
- Building Automation
- HVAC control
- Energy Management systems
- Industrial process control.
Conclusion.
We hope you have found this Touch Sensor Toggle Relay Circuit very useful. If you feel any difficulty in making it feel free to ask anything in the comment section.