Here we have created a simple webpage with esp8266 which has different color codes each color have a unique code for RGB (Red, Green & Blue) LED. RBG LED we used here is common Anode which means +ve of all LED’s is common and LED glows from dark to light depending on the value received via webpage by the user.
PCBWay commits to meeting the needs of its customers from different industries in terms of quality, delivery, cost-effectiveness, and any other demanding requests. As one of the most experienced PCB manufacturers in China. They have an open source community, people can share their design projects with each other. Moreover, every time people place an order for your board, PCBWay will donate 10% of the cost of PCB to you, for your contribution to the Open Source Community.
Hardware Required
RGB LED Circuit
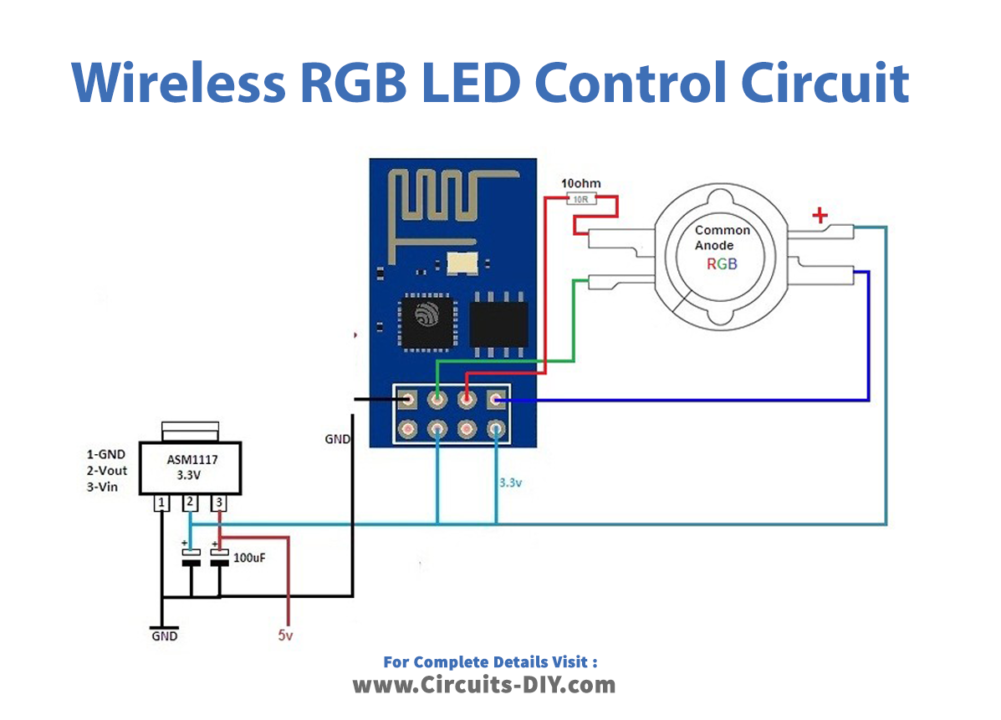
RGB LED Code
#include <ESP8266WiFi.h>
#include <DNSServer.h>
#include <ESP8266WebServer.h>
const char *ssid = "RGB";
//Uncomment below line if you wish to set a password for ESP wifi network...
// const char *password = "87654321";
const byte DNS_PORT = 53;
IPAddress apIP(192, 168, 1, 1); //IP address of your ESP
DNSServer dnsServer;
ESP8266WebServer webServer(80);
//Webpage html Code
String webpage = ""
"<!DOCTYPE html><html><head><title>RGB control eTechPath.com</title><meta name='mobile-web-app-capable' content='yes' />"
"<meta name='viewport' content='width=device-width' /></head><body style='margin: 0px; padding: 0px;'>"
"<canvas id='colorspace'></canvas>"
"</body>"
"<script type='text/javascript'>"
"(function () {"
" var canvas = document.getElementById('colorspace');"
" var ctx = canvas.getContext('2d');"
" function drawCanvas() {"
" var colours = ctx.createLinearGradient(0, 0, window.innerWidth, 0);"
" for(var i=0; i <= 360; i+=10) {"
" colours.addColorStop(i/360, 'hsl(' + i + ', 100%, 50%)');"
" }"
" ctx.fillStyle = colours;"
" ctx.fillRect(0, 0, window.innerWidth, window.innerHeight);"
" var luminance = ctx.createLinearGradient(0, 0, 0, ctx.canvas.height);"
" luminance.addColorStop(0, '#ffffff');"
" luminance.addColorStop(0.05, '#ffffff');"
" luminance.addColorStop(0.5, 'rgba(0,0,0,0)');"
" luminance.addColorStop(0.95, '#000000');"
" luminance.addColorStop(1, '#000000');"
" ctx.fillStyle = luminance;"
" ctx.fillRect(0, 0, ctx.canvas.width, ctx.canvas.height);"
" }"
" var eventLocked = false;"
" function handleEvent(clientX, clientY) {"
" if(eventLocked) {"
" return;"
" }"
" function colourCorrect(v) {"
" return Math.round(1023-(v*v)/64);"
" }"
" var data = ctx.getImageData(clientX, clientY, 1, 1).data;"
" var params = ["
" 'r=' + colourCorrect(data[0]),"
" 'g=' + colourCorrect(data[1]),"
" 'b=' + colourCorrect(data[2])"
" ].join('&');"
" var req = new XMLHttpRequest();"
" req.open('POST', '?' + params, true);"
" req.send();"
" eventLocked = true;"
" req.onreadystatechange = function() {"
" if(req.readyState == 4) {"
" eventLocked = false;"
" }"
" }"
" }"
" canvas.addEventListener('click', function(event) {"
" handleEvent(event.clientX, event.clientY, true);"
" }, false);"
" canvas.addEventListener('touchmove', function(event){"
" handleEvent(event.touches[0].clientX, event.touches[0].clientY);"
"}, false);"
" function resizeCanvas() {"
" canvas.width = window.innerWidth;"
" canvas.height = window.innerHeight;"
" drawCanvas();"
" }"
" window.addEventListener('resize', resizeCanvas, false);"
" resizeCanvas();"
" drawCanvas();"
" document.ontouchmove = function(e) {e.preventDefault()};"
" })();"
"</script></html>";
void handleRoot()
{
// Serial.println("handle root..");
String red = webServer.arg(0); // read RGB arguments
String green = webServer.arg(1); // read RGB arguments
String blue = webServer.arg(2); // read RGB arguments
//for common anode
analogWrite(0, red.toInt());
analogWrite(2, green.toInt());
analogWrite(3, blue.toInt());
//for common cathode
//analogWrite(0,1023 - red.toInt());
//analogWrite(2,1023 - green.toInt());
//analogWrite(3,1023 - blue.toInt());
webServer.send(200, "text/html", webpage);
}
void setup()
{
pinMode(0, OUTPUT);
pinMode(2, OUTPUT);
pinMode(3, OUTPUT);
analogWrite(0, 1023);
analogWrite(2, 1023);
analogWrite(3, 1023);
delay(1000);
WiFi.mode(WIFI_AP);
WiFi.softAPConfig(apIP, apIP, IPAddress(255, 255, 255, 0));
WiFi.softAP(ssid);
dnsServer.start(DNS_PORT, "rgb", apIP);
webServer.on("/", handleRoot);
webServer.begin();
testRGB();
}
void loop()
{
dnsServer.processNextRequest();
webServer.handleClient();
}
void testRGB()
{
// fade in and out of Red, Green, Blue
analogWrite(0, 1023); // Red off
analogWrite(2, 1023); // Green off
analogWrite(3, 1023); // Blue off
fade(0); // Red fade effect
fade(2); // Green fade effect
fade(3); // Blue fade effect
}
void fade(int pin)
{
for (int u = 0; u < 1024; u++)
{
analogWrite(pin, 1023 - u);
delay(1);
}
for (int u = 0; u < 1024; u++)
{
analogWrite(pin, u);
delay(1);
}
}
Working Explanation
- Connect the final circuit as shown in the above diagram and power it up using a 5v battery or wall adapter.
- ESP module will boot up and the LED light will show a fade effect in all three colors at startup.
- Then open your device WiFi in discovery mode and you will see a new WiFi access point, named RGB in the discovery list.
- Connect that WiFi access point, Open any web browser on that device, and open IP address 192.168.1.1, that’s it, you will see a colorful RGB control screen to control your wireless RGB LED.